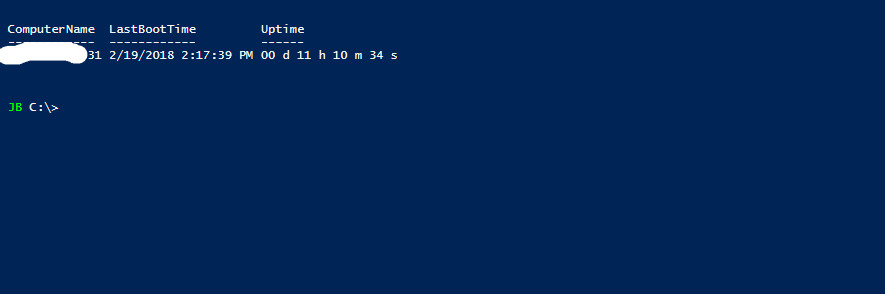
Computer uptime is an important statistic in systems management. Here are several of the ways we can determine system uptime for a computer (note that this list is by no means exhaustive):
1. The Task Manager’s Performance tab displays the computer uptime as days, hours, minutes and seconds.
2. The Systeminfo command-line tool displays the computer’s last boot time.
3. The most recent event ID 6005 in the computer’s System event log records the computer’s last boot time.
4. The WMI Win32_OperatingSystem class has a LastBootUpTime property that contains the computer’s last boot time.
Each of these techniques has their pros and cons. For example, the Task Manager provides quick visibility for a single computer’s uptime, and the event log contains additional information around the 6005 event that may provide insight about a system failure. The first three choices are probably not the best for automation purposes (e.g., querying a list of servers for uptime), so let’s look to WMI. First, though, we need to take a look at how WMI returns uptime information.
Computer uptime is an important statistic in systems management. Here are several of the ways we can determine system uptime for a computer (note that this list is by no means exhaustive):
1. The Task Manager’s Performance tab displays the computer uptime as days, hours, minutes and seconds.
2. The Systeminfo command-line tool displays the computer’s last boot time.
3. The most recent event ID 6005 in the computer’s System event log records the computer’s last boot time.
4. The WMI Win32_OperatingSystem class has a LastBootUpTime property that contains the computer’s last boot time.
Each of these techniques has their pros and cons. For example, the Task Manager provides quick visibility for a single computer’s uptime, and the event log contains additional information around the 6005 event that may provide insight about a system failure. The first three choices are probably not the best for automation purposes (e.g., querying a list of servers for uptime), so let’s look to WMI. First, though, we need to take a look at how WMI returns uptime information.
Using WMI to Get Computer Uptime
There are several ways we can retrieve a computer’s uptime using WMI. One of the most obvious ways, prior to Windows PowerShell, was to use the WMIC command. For example:
wmic path Win32_OperatingSystem get LastBootUpTime
This command retrieves the LastBootUpTime property of the Win32_OperatingSystem class instance on the computer. In PowerShell, we would use the Get-WmiObject cmdlet rather than WMIC:
Get-WmiObject Win32_OperatingSystem | Select-Object LastBootUpTime
Try these commands yourself, and you will see that the output is not necessarily “user-friendly” because the date and time expressed in the LastBootUpTime property is formatted as a CIM (Common Information Model) datetime string. For example:
20160512154836.125599-360
This datetime string translates to May 12, 2016, 15:48:36 (3:48pm). 125599 is the number of microseconds (we’ll ignore those), and the -360 represents the number of minutes offset from GMT. In this example, -360 means the time is 6 hours behind GMT. (Similarly, +180 would mean “3 hours ahead of GMT.”)
To make the CIM datetime string more usable, we will need to convert it into a usable date (a DateTime object). Fortunately, PowerShell makes this simple: The ToDateTime static method of the System.Management.ManagementDateTimeConverter .NET class converts the CIM datetime string to a DateTime object we can use more easily in PowerShell. Figure 1 shows an example.
#requires -version 2 <# .SYNOPSIS Outputs the last bootup time and uptime for one or more computers. .DESCRIPTION Outputs the last bootup time and uptime for one or more computers. .PARAMETER ComputerName One or more computer names. The default is the current computer. Wildcards are not supported. .PARAMETER Credential Specifies credentials that have permission to connect to the remote computer. This parameter is ignored for the current computer. .OUTPUTS PSObjects containing the computer name, the last bootup time, and the uptime. #> [CmdletBinding()] param( [parameter(ValueFromPipeline=$true,ValueFromPipelineByPropertyName=$true)] $ComputerName, [System.Management.Automation.PSCredential] $Credential ) begin { function Out-Object { param( [System.Collections.Hashtable[]] $hashData ) $order = @() $result = @{} $hashData | ForEach-Object { $order += ($_.Keys -as [Array])[0] $result += $_ } New-Object PSObject -Property $result | Select-Object $order } function Format-TimeSpan { process { "{0:00} d {1:00} h {2:00} m {3:00} s" -f $_.Days,$_.Hours,$_.Minutes,$_.Seconds } } function Get-Uptime { param( $computerName, $credential ) # In case pipeline input contains ComputerName property if ( $computerName.ComputerName ) { $computerName = $computerName.ComputerName } if ( (-not $computerName) -or ($computerName -eq ".") ) { $computerName = [Net.Dns]::GetHostName() } $params = @{ "Class" = "Win32_OperatingSystem" "ComputerName" = $computerName "Namespace" = "root\CIMV2" } if ( $credential ) { # Ignore -Credential for current computer if ( $computerName -ne [Net.Dns]::GetHostName() ) { $params.Add("Credential", $credential) } } try { $wmiOS = Get-WmiObject @params -ErrorAction Stop } catch { Write-Error -Exception (New-Object $_.Exception.GetType().FullName ` ("Cannot connect to the computer '$computerName' due to the following error: '$($_.Exception.Message)'", $_.Exception)) return } $lastBootTime = [Management.ManagementDateTimeConverter]::ToDateTime($wmiOS.LastBootUpTime) Out-Object ` @{"ComputerName" = $computerName}, @{"LastBootTime" = $lastBootTime}, @{"Uptime" = (Get-Date) - $lastBootTime | Format-TimeSpan} } } process { if ( $ComputerName ) { foreach ( $computerNameItem in $ComputerName ) { Get-Uptime $computerNameItem $Credential } } else { Get-Uptime "." } } Reference: http://www.itprotoday.com/management-mobility/getting-computer-uptime-using-powershell
Hi there just wanted to give you a quick heads up. The text in your content seem to be running off the screen in Firefox. I’m not sure if this is a formatting issue or something to do with internet browser compatibility but I thought I’d post to let you know. The design look great though! Hope you get the problem resolved soon. Cheers
8ks6ty
l80e0u
czdqfk
mwllsj
54f05m